Posted by mitch
on October 31, 2012
software
How many times have you seen the UPS landing page below?
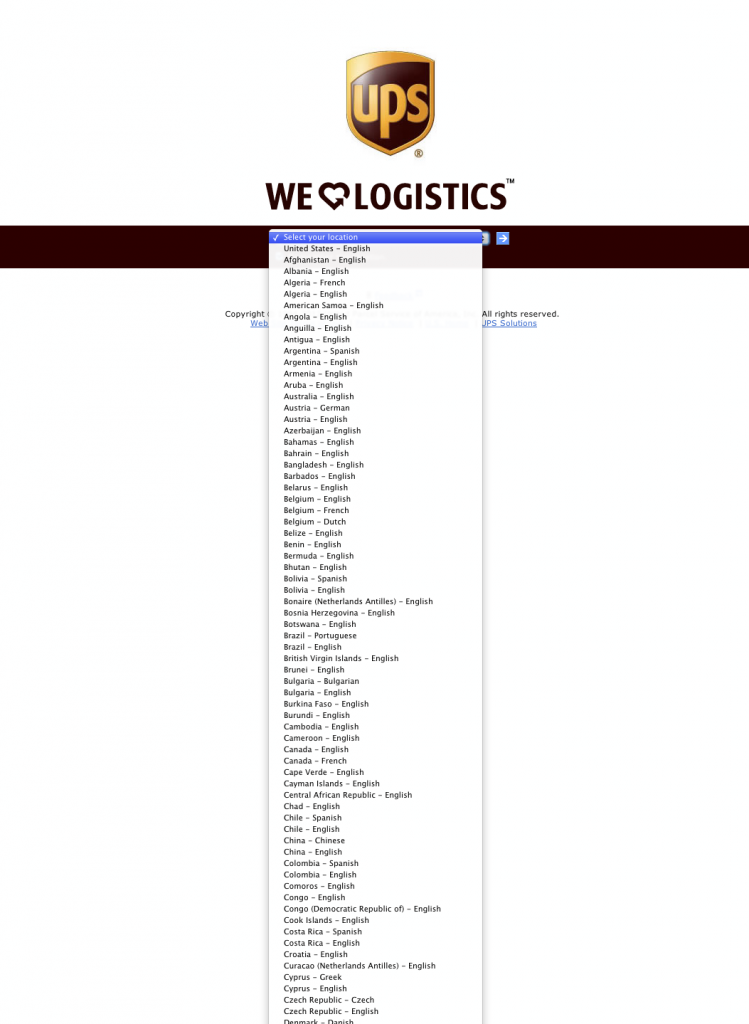
Why is it that neither FedEx nor UPS know about geolocation of IP addresses? Even if geolocation was used just as a hint or an obvious way was provided to change it if the geolocation went wrong, far fewer folks would hit these screens. And after you make a selection, the UPS site just sits there and does nothing until you click the small blue botton.
I hate software that makes me deal with pop quizzes and stupid modal dialogs. Hey, where are you? Would you like fast or small? Want to update now? Restart Firefox now? Hey, you will need to reboot after you install this, OK? Downloading this RPM will use 30 KB of disk space, sound good? Really quit? There’s an item on your calendar coming up in 10 minutes, you won’t be able to click anywhere in Gmail until you click this OK button… on all of your computers. I couldn’t copy that to the clipboard. Send a bug report to Apple? This document has ColorSync profile 1, and this other one has ColorSync profile 2. Would you like me to alert when submitting a form to an unencrypted site? There was an error with your request, try again later. All the downloadable content has downloaded. I am going to go ahead and join you to the unsecured network ‘linksys’. Hey, couldn’t backup this computer. Holy shit, there’s new software available, I don’t care if you’re watching a movie fullscreen (iTunes + Notification Center). Psst, mind if I phone home real quick? Oh, by the way, the iTunes terms and conditions have changed and I suddenly can’t remember your credit card number.
Who wouldn’t hate a machine that talks to you this way, all day, every day? I am trying to get some work done here, and you’re telling me all this out-of-band shit and very little of it is useful to me right now.
Please stop asking me questions. I don’t know what the answer is and you’re interrupting my train of thought, which I value far more than pondering any of the above questions.
Tags: modal, rant, stupid, ui, ux
Posted by mitch
on October 31, 2012
hardware,
projects,
software
There are lots of examples of how to use the Ethernet Wiznet chips with Arduino, whether as Ethernet shields or as Ethernet Arduinos on a single board. Unfortunately, most of these examples hard-code the hardware (MAC) address, which can make things painful if you’re building more than one device and running them on the same network.
The code snippet below is a more convenient approach. You can setup a prefix (DEADBEEF in the example below) for the hardware address and the last two bytes are set randomly on first boot. The hardware address is stored in EEPROM (7 bytes are needed, 1 for a flag indicating that the next 6 bytes are properly populated).
The bytes->String conversion below is a bit ugly but I didn’t think I wanted the overhead of sprint in this. It is probably not worth the trade off. (0x30 is ‘0’ and 0x39 is ‘9’. Adding 0x07 skips over some ASCII characters to ‘A’.)
Some serious caveats: There’s only two bytes of randomness here. You might want more. Ideally you would have a manufacturing process, but if you’re just building six devices, who cares? Clearly you would never use this approach in a production environment, but it’s easier than changing the firmware for every device in a hobby environment. You could also use a separate program to write the EEPROM hardware address and keep this “manufacturing junk” out of your main firmware. These issues aside, my main requirement is convenience: I want to be able to burn a single image onto a new board and be up and running immediately without having to remember other steps. Convenience influences repeatability.
#include <Ethernet.h>
#include <EEPROM.h>
// This is a template address; the last two bytes will be randomly
// generated on the first boot and filled in. On later boots, the
// bytes are pulled from EEPROM.
byte NETWORK_HW_ADDRESS[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0x00, 0x00};
String NETWORK_HW_ADDRESS_STRING = "ERROR_NOT_FILLED_IN";
// These are commented out so that this code will not compile
// without the reader modifying these lines. If you are using
// EEPROM code in your program already, you need to put the
// network address somewhere that doesn't collide with existing use.
//#define EEPROM_INIT_FLAG_ADDR 0
//#define EEPROM_HWADDR_START_ADDR 1
// Call this from your setup routine (see below)
void
initEthernetHardwareAddress() {
int eeprom_flag = EEPROM.read(EEPROM_INIT_FLAG_ADDR);
int i;
Serial.print("EEPROM flag is " + String(eeprom_flag));
if (eeprom_flag != 0xCC) {
NETWORK_HW_ADDRESS[4] = random(255);
NETWORK_HW_ADDRESS[5] = random(255);
// write it out.
Serial.println("Writing generated hwaddr to EEPROM...");
for (i = 0; i < 6; i++) {
EEPROM.write(EEPROM_HWADDR_START_ADDR + i + 1,
NETWORK_HW_ADDRESS[i]);
}
EEPROM.write(EEPROM_INIT_FLAG_ADDR, 0xCC);
} else {
Serial.print("Reading network hwaddr from EEPROM...");
for (i = 0; i < 6; i++) {
NETWORK_HW_ADDRESS[i] =
EEPROM.read(EEPROM_HWADDR_START_ADDR + i + 1);
}
}
char hw_string[13];
hw_string[12] = '\0';
for (i = 0; i < 6; i++) {
int j = i * 2;
int the_byte = NETWORK_HW_ADDRESS[i];
int first_part = (the_byte & 0xf0) >> 4;
int second_part = (the_byte & 0x0f);
first_part += 0x30;
second_part += 0x30;
if (first_part > 0x39) {
first_part += 0x07;
}
if (second_part > 0x39) {
second_part += 0x07;
}
hw_string[j] = first_part;
hw_string[j + 1] = second_part;
}
NETWORK_HW_ADDRESS_STRING = String(hw_string);
Serial.println("NETWORK_ADDR = " + NETWORK_HW_ADDRESS_STRING);
}
void
setup() {
// first call the usual Serial.begin and so forth...
// setup the Ethernet hwaddr before you start using networking
initEthernetHardwareAddress();
int dhcp_worked = Ethernet.begin(NETWORK_HW_ADDRESS);
// ...
}